javascript:(function(){
let textToPaste = "MY SNIPPET";
let field = document.activeElement;
if (field && (field.tagName === "INPUT" || field.tagName === "TEXTAREA")) {
field.value += textToPaste;
field.dispatchEvent(new Event('input', { bubbles: true }));
} else {
navigator.clipboard.writeText(textToPaste).then(() => {
alert("Text copied to clipboard! Paste it anywhere.");
}).catch(err => {
alert("Failed to copy text: " + err);
});
}
})();
If there’s an active text field, it pastes the text directly. Otherwise, it copies it to the clipboard so the user can manually paste it anywhere.
No alert version (you need to press CTRL+V after):
javascript:(function(){
let textToPaste = "$x Vingerafdruk Controlleren ";
let field = document.activeElement;
if (field && (field.tagName === "INPUT" || field.tagName === "TEXTAREA")) {
field.value += textToPaste;
field.dispatchEvent(new Event('input', { bubbles: true }));
} else {
navigator.clipboard.writeText(textToPaste).catch(() => {});
}
})();
Selection and autopasting
javascript:(async function() {
const snippets = [
"string of text one",
"string of text two",
"string of text three",
"string of text four"
];
async function insertText(text) {
let activeElement = document.activeElement;
if (activeElement && (activeElement.isContentEditable || ['INPUT', 'TEXTAREA'].includes(activeElement.tagName))) {
let start = activeElement.selectionStart;
let end = activeElement.selectionEnd;
let value = activeElement.value;
activeElement.value = value.slice(0, start) + text + value.slice(end);
activeElement.selectionStart = activeElement.selectionEnd = start + text.length;
activeElement.focus();
} else {
try {
await navigator.clipboard.writeText(text);
const pastePermission = await navigator.permissions.query({ name: "clipboard-read" });
if (pastePermission.state === "granted" || pastePermission.state === "prompt") {
let pasteText = await navigator.clipboard.readText();
document.execCommand('insertText', false, pasteText);
}
} catch (err) {
alert("Snippet copied to clipboard! Paste manually with Ctrl+V.");
}
}
}
document.addEventListener('click', function(event) {
event.preventDefault();
let menu = document.createElement('div');
menu.style.position = 'fixed';
menu.style.left = event.clientX + 'px';
menu.style.top = event.clientY + 'px';
menu.style.background = '#fff';
menu.style.border = '1px solid #333';
menu.style.boxShadow = '2px 2px 10px rgba(0, 0, 0, 0.2)';
menu.style.padding = '5px';
menu.style.zIndex = '9999';
menu.style.fontSize = '14px';
menu.style.color = '#000';
menu.style.minWidth = '220px';
snippets.forEach(text => {
let btn = document.createElement('button');
btn.textContent = text;
btn.style.display = 'block';
btn.style.width = '100%';
btn.style.textAlign = 'left';
btn.style.border = 'none';
btn.style.background = 'white';
btn.style.padding = '8px';
btn.style.cursor = 'pointer';
btn.style.fontSize = '14px';
btn.onmouseover = () => btn.style.background = '#ddd';
btn.onmouseout = () => btn.style.background = 'white';
btn.onclick = async () => {
await insertText(text);
document.body.removeChild(menu);
};
menu.appendChild(btn);
});
document.body.appendChild(menu);
function removeMenu() {
if (document.body.contains(menu)) {
document.body.removeChild(menu);
}
document.removeEventListener('click', removeMenu);
document.removeEventListener('keydown', keyClose);
}
function keyClose(e) {
if (e.key === 'Escape') {
removeMenu();
}
}
setTimeout(() => {
document.addEventListener('click', removeMenu);
document.addEventListener('keydown', keyClose);
}, 100);
}, { once: true });
})();
Selection autopasting and cute:
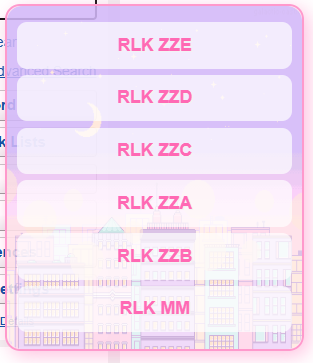
javascript:(async function () { const snippets = [
"string of text one",
"string of text two",
"string of text three",
"string of text four"
]; async function insertText(text) { let activeElement = document.activeElement; if (activeElement && (activeElement.isContentEditable || ["INPUT", "TEXTAREA"].includes(activeElement.tagName))) { let start = activeElement.selectionStart; let end = activeElement.selectionEnd; let value = activeElement.value; activeElement.value = value.slice(0, start) + text + value.slice(end); activeElement.selectionStart = activeElement.selectionEnd = start + text.length; activeElement.focus(); } else { try { await navigator.clipboard.writeText(text); const pastePermission = await navigator.permissions.query({ name: "clipboard-read" }); if (pastePermission.state === "granted" || pastePermission.state === "prompt") { let pasteText = await navigator.clipboard.readText(); document.execCommand("insertText", false, pasteText); } } catch (err) { console.error("Clipboard access denied."); } } } document.addEventListener("click", function (event) { event.preventDefault(); let menu = document.createElement("div"); menu.style.position = "fixed"; menu.style.left = event.clientX + "px"; menu.style.top = event.clientY + "px"; menu.style.background = "rgba(255, 230, 242, 0.8)"; menu.style.border = "2px solid #ff99cc"; menu.style.boxShadow = "4px 4px 15px rgba(255, 105, 180, 0.4)"; menu.style.padding = "8px"; menu.style.zIndex = "9999"; menu.style.fontSize = "14px"; menu.style.color = "#ff1493"; menu.style.minWidth = "220px"; menu.style.borderRadius = "12px"; menu.style.fontFamily = "Arial, sans-serif"; menu.style.overflow = "hidden"; menu.style.position = "absolute"; let img = document.createElement("img"); img.src = "https://docs.marisabel.nl/wp-content/uploads/2025/03/tumblr_06b12b250c154600c4e99f0b9c7be739_c1af3900_400.gif"; img.style.position = "absolute"; img.style.top = "0"; img.style.left = "0"; img.style.width = "100%"; img.style.height = "100%"; img.style.opacity = "0.4"; img.style.borderRadius = "12px"; img.style.zIndex = "-1"; menu.appendChild(img); snippets.forEach((text) => { let btn = document.createElement("button"); btn.textContent = text; btn.style.display = "block"; btn.style.width = "100%"; btn.style.textAlign = "center"; btn.style.border = "none"; btn.style.background = "rgba(255, 255, 255, 0.7)"; btn.style.padding = "10px"; btn.style.margin = "5px 0"; btn.style.cursor = "pointer"; btn.style.fontSize = "14px"; btn.style.borderRadius = "8px"; btn.style.color = "#ff69b4"; btn.style.fontWeight = "bold"; btn.style.transition = "background 0.3s, color 0.3s"; btn.onmouseover = () => { btn.style.background = "#ffff99"; btn.style.color = "#ff4500"; }; btn.onmouseout = () => { btn.style.background = "rgba(255, 255, 255, 0.7)"; btn.style.color = "#ff69b4"; }; btn.onclick = async () => { await insertText(text); document.body.removeChild(menu); }; menu.appendChild(btn); }); document.body.appendChild(menu); function removeMenu() { if (document.body.contains(menu)) { document.body.removeChild(menu); } document.removeEventListener("click", removeMenu); document.removeEventListener("keydown", keyClose); } function keyClose(e) { if (e.key === "Escape") { removeMenu(); } } setTimeout(() => { document.addEventListener("click", removeMenu); document.addEventListener("keydown", keyClose); }, 100); }, { once: true }); })();
Comments are closed.